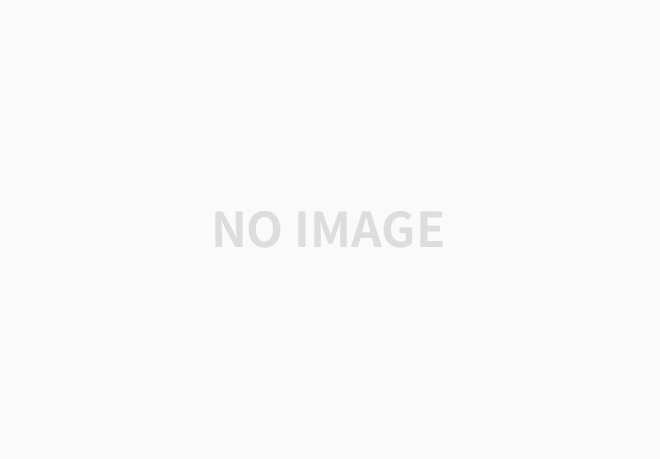
- Apache POI, 마이크로소프트 오피스 지원 라이브러리
Apache POI는 아파치 소프트웨어 재단에서 만든 라이브러리로서 마이크로소프트 오피스 파일 포맷을 순수 자바 언어로서 읽고 쓰는 기능을 제공한다.
- MVN Repository
https://mvnrepository.com/artifact/org.apache.poi/poi
https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml
- settings.gradle
implementation 'org.apache.poi:poi:5.2.3'
implementation 'org.apache.poi:poi-ooxml:5.2.3'
- ReadExcel.java
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.util.Iterator;
public class ReadExcel {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream("src/data/서울특별시_관악구_폐형광등 및 폐건전지 분리수거함_20220809.xlsx");
//Create Workbook instance holding reference to .xlsx file
XSSFWorkbook workbook = new XSSFWorkbook(file);
//Get first/desired sheet from the workbook
XSSFSheet sheet = workbook.getSheetAt(0);
//Iterate through each rows one by one
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
//For each row, iterate through all the columns
Iterator<Cell> cellIterator = row.cellIterator();
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
//Check the cell type and format accordingly
switch (cell.getCellType()) {
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
default:
throw new IllegalStateException("Unexpected value: " + cell.getCellType());
}
}
System.out.println("");
}
file.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
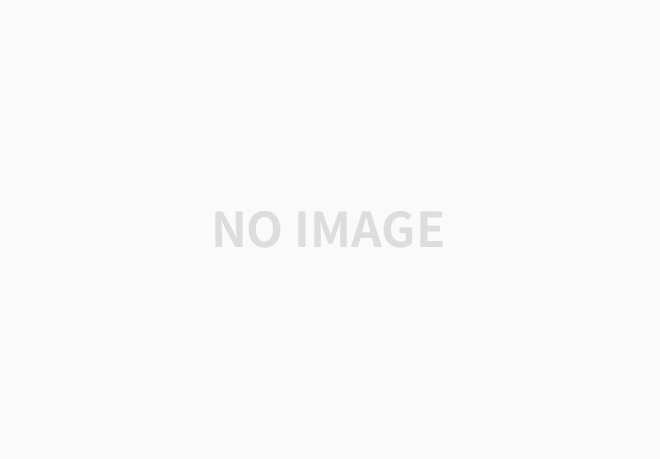
- .xls, .xlsx 파일 처리 방법
FileInputStream file = new FileInputStream(templateFile);
if (templateFile.getAbsolutePath().endsWith("xlsx")) {
workbook = new XSSFWorkbook(file);
} else if (templateFile.getAbsolutePath().endsWith("xls")) {
workbook = new HSSFWorkbook(file);
}
- 참고자료
Apache POI - Read and Write Excel File in Java
Learn to read and write excel files, add and evaluate formula cells and add color formatting in Java using Apache POI with examples.
howtodoinjava.com